Layered Pattern
Layered Patternμ΄λ π
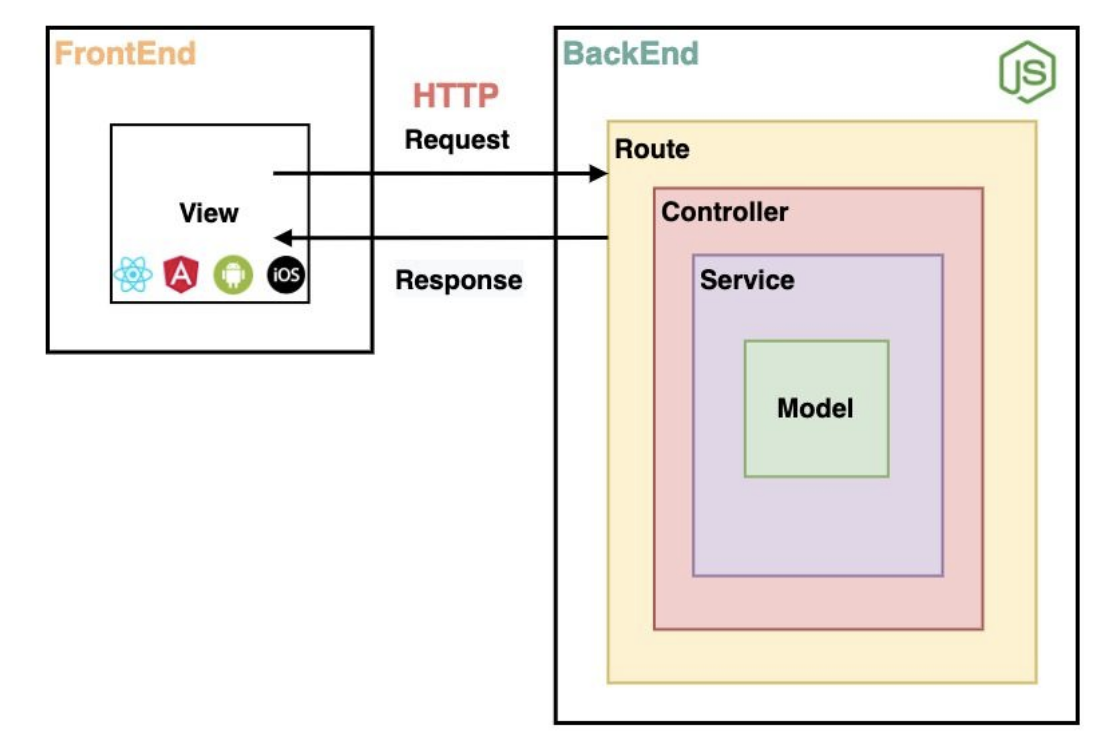
Route, Controller, Service, Model κ°κ°μ λ μ΄μ΄κ° νλμ ν΄λμ΄μ μν μ μλ―Ένλ€.
- ν° λ°μ€μμ μμ λ°μ€λ‘ κ° μλ‘ λ λ°μ΄ν°λ₯Ό λ€λ£¨λ λ‘μ§(λ°μ΄ν°λ² μ΄μ€ μ κ·Όνλ λ‘μ§)μ κ·Όμ νκ² λλ€.
- λν, κ°κ°μ λ μ΄μ΄λ μ€λ‘μ§ λ°λ‘ μλμ μλ λ μ΄μ΄μλ§ μμ‘΄νκ² λλ€.
- Route β Controller
- Controller β Service
- Service β Model
μλ₯Όλ€μ΄, Route λ Service λ‘μ§μ μ ν λͺ¨λ₯΄κ³ μμ κ΄μ¬ μ‘°μ°¨ νμ§ μλλ€. λ°λΌμ, Service λ‘μ§μ λ³κ²½ν΄λ Route μ Controller μ μ½λλ λ°λ νμκ° μλ€.
μ¦ λ€μκ³Ό κ°μ μν©μμ μ μ°νκ² λμ²ν μ μλ€λ μλ―Έμ΄λ€.
λλλ‘, μλΉμ€λ₯Ό ꡬννλ€κ° RDBMS(κ΄κ³ν λ°μ΄ν° λ² μ΄μ€) β NoSQL(ex. mongoDB) λ‘ μ΄μ νλ κ²½μ°κ° μλλ°, Routeμ Controller μ λ‘μ§μ μ ν λ°λμ§ μμμ±λ‘ λ°μ΄ν°λ₯Ό λ€λ£¨λ Service μ Model μ λ‘μ§λ§ λ³κ²½ ν΄ μ£Όλ©΄ λλ€.
μ€μ΅
model
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
const productDetail = async productId => {
const detail = await prisma.$queryRaw`
SELECT
products.id,
eng_name,
price,
is_new,
is_main,
quantity,
sale_rate,
categories.name as cate_name,
subcategories.name as subcate_name,
url,
images.id as image_id
FROM
products
JOIN
categories on category_id = categories.id
JOIN
images on products.id = images.product_id
JOIN
subcategories on subcategories.id = subcategory_id
WHERE
products.id = ${productId};
`;
return detail;
};
export default { productDetail };
service
import { productDetailDao } from '../models';
const productDetail = async productId => {
const detail = await productDetailDao.productDetail(productId);
const tmp = detail[0];
tmp.url = detail.map((e, i) => {
return { id: detail[i]['image_id'], image: detail[i].url };
});
return detail[0];
};
export default { productDetail };
controller
import { productDetailServices } from '../services';
const productDetail = async (req, res) => {
try {
const { productId } = req.params;
const detail = await productDetailServices.productDetail(productId);
res.status(200).send(detail);
} catch (err) {
console.log(err);
res.status(500).send(err);
}
};
export default { productDetail };
μ²μμλ μ΄λ° λͺ¨λνλ₯Ό μνλμ§ μ΄ν΄κ° λμ§μμλλ°
νμ μ νλκ³Όμ μμ μ΄ κ³Όμ μ΄ μ νμνκ°λ₯Ό μμ£Ό κΉμ΄ κΉ¨λ¬μλ€.
λ§μ½ λͺ¨λνλ₯Ό νμ§μμλ€λ©΄ νμλ€μ΄ μμ±ν μ½λλ₯Ό λΆμνλλ° μμ΄μ μλΉν μκ°μ΄ κ±Έλ Έμκ²μ΄λ€.
λ€νν layered patternμ μ¬μ©νλ νμλ€μ΄ 무μμ μκ°νλ©° μ½λλ₯Ό μ§κ³
μ΄λ€ APIλ₯Ό λ§λ€μλμ§ νλμ μμμμκ³
μμ λν μ΄λλΆλΆμ μμ ν΄μΌνλμ§ λ°λ‘ μμμμλ€.