GraphQL-yoga 간단한 실습2
실습해보기2
mutation을 사용해보자
graphql/schema.graphql
type Movie {
id: Int!
name: String!
score: Int!
}
type Query {
movies: [Movie]!
movie(id: Int!): Movie
}
type Mutation {
addMovie(name: String!, score: Int!): Movie!
deleteMovie(id: Int!): Boolean!
}
graphql/resolvers.js
let movies = [
{ id: 0, name: '하울의 움직이는 성', score: 3 },
{ id: 1, name: '백설공주', score: 8 },
{ id: 2, name: '빨간머리 앤', score: 99 },
{ id: 3, name: '인어공주', score: 2 },
];
const getMovies = () => movies;
const getById = (id) => {
const filterMovies = movies.filter((movie) => (movie.id = id));
return filterMovies[0];
};
const deleteMovie = (id) => {
const cleanedMovies = movies.filter((movie) => movie.id !== id);
if (movies.length > cleanedMovies.length) {
movies = cleanedMovies;
return true;
} else {
return false;
}
};
const addMovie = (name, score) => {
const newMovie = {
id: movies.length + 1,
name,
score,
};
movies.push(newMovie);
return newMovie;
};
const resolvers = {
Query: {
movies: () => getMovies(),
movie: (_, { id }) => getById(id),
},
Mutation: {
addMovie: (_, { name, score }) => addMovie(name, score),
deleteMovie: (_, { id }) => deleteMovie(id),
},
};
export default resolvers;
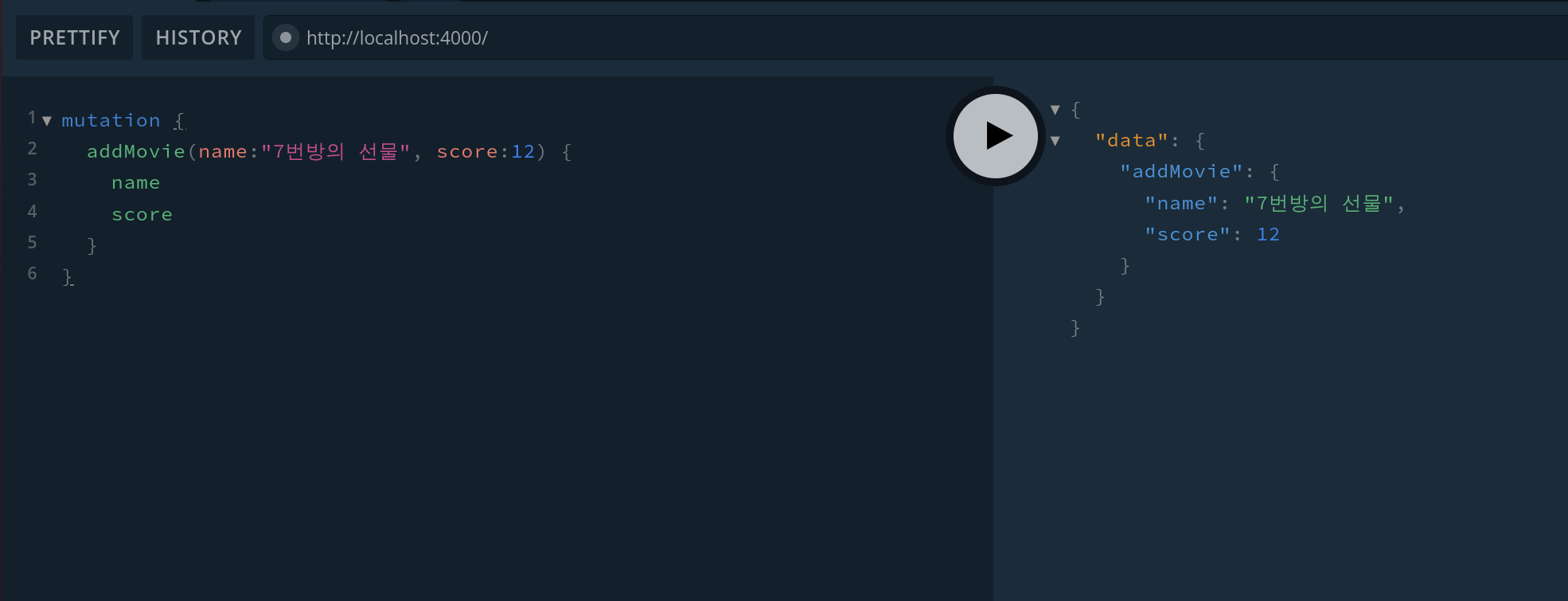
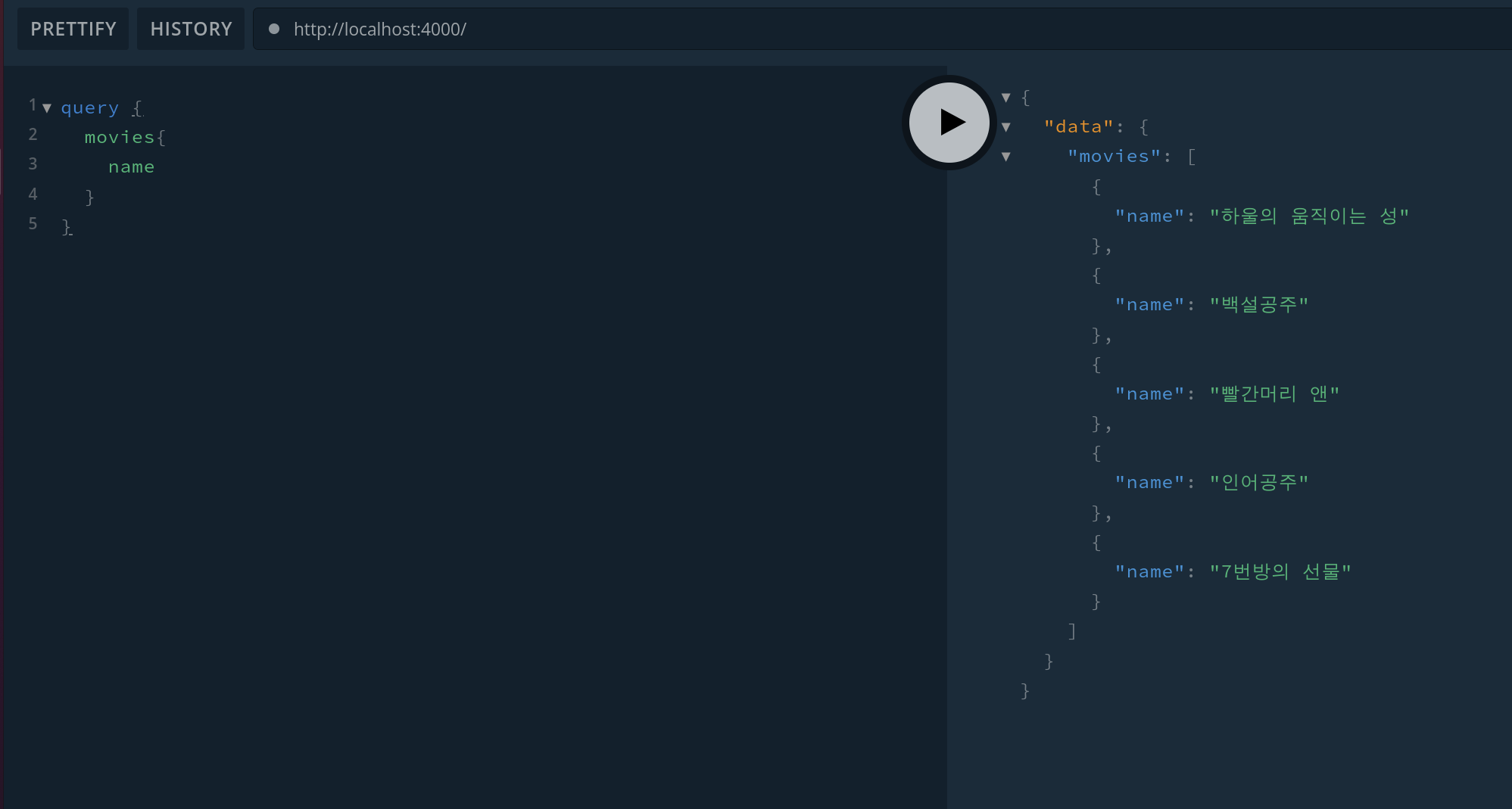
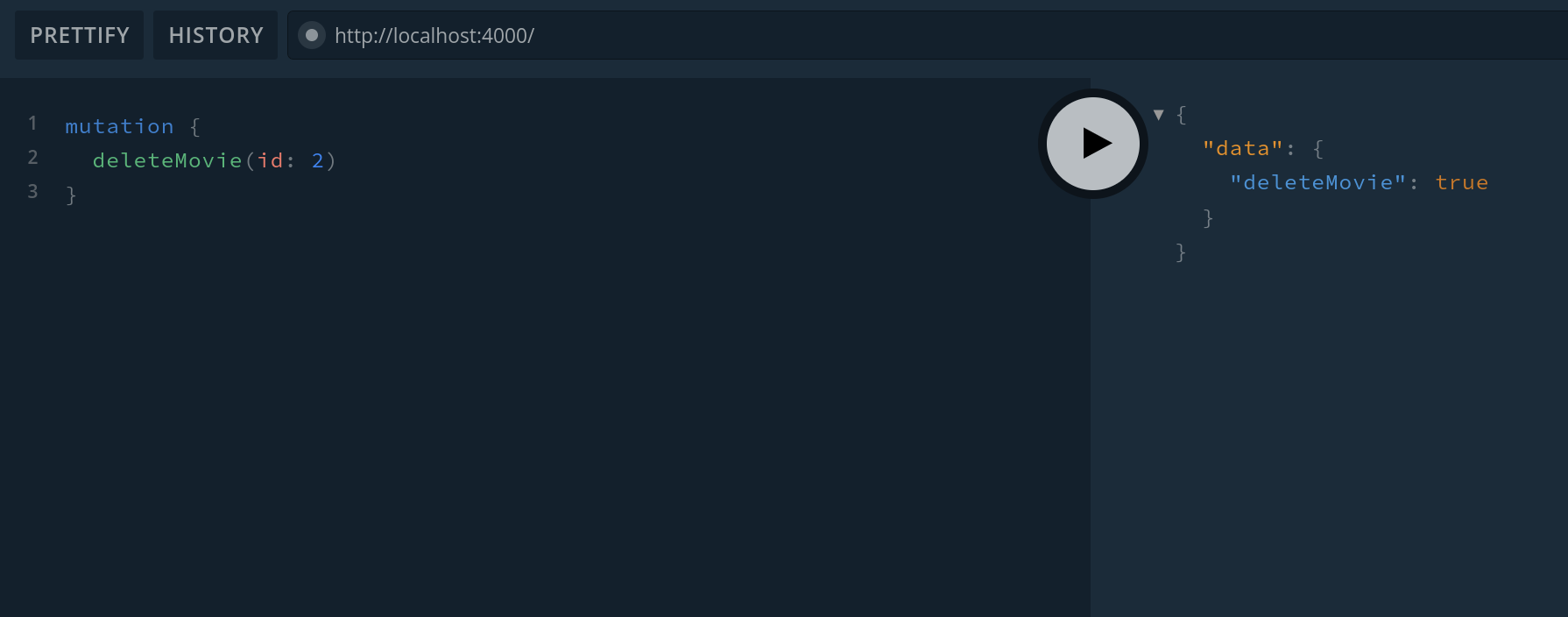
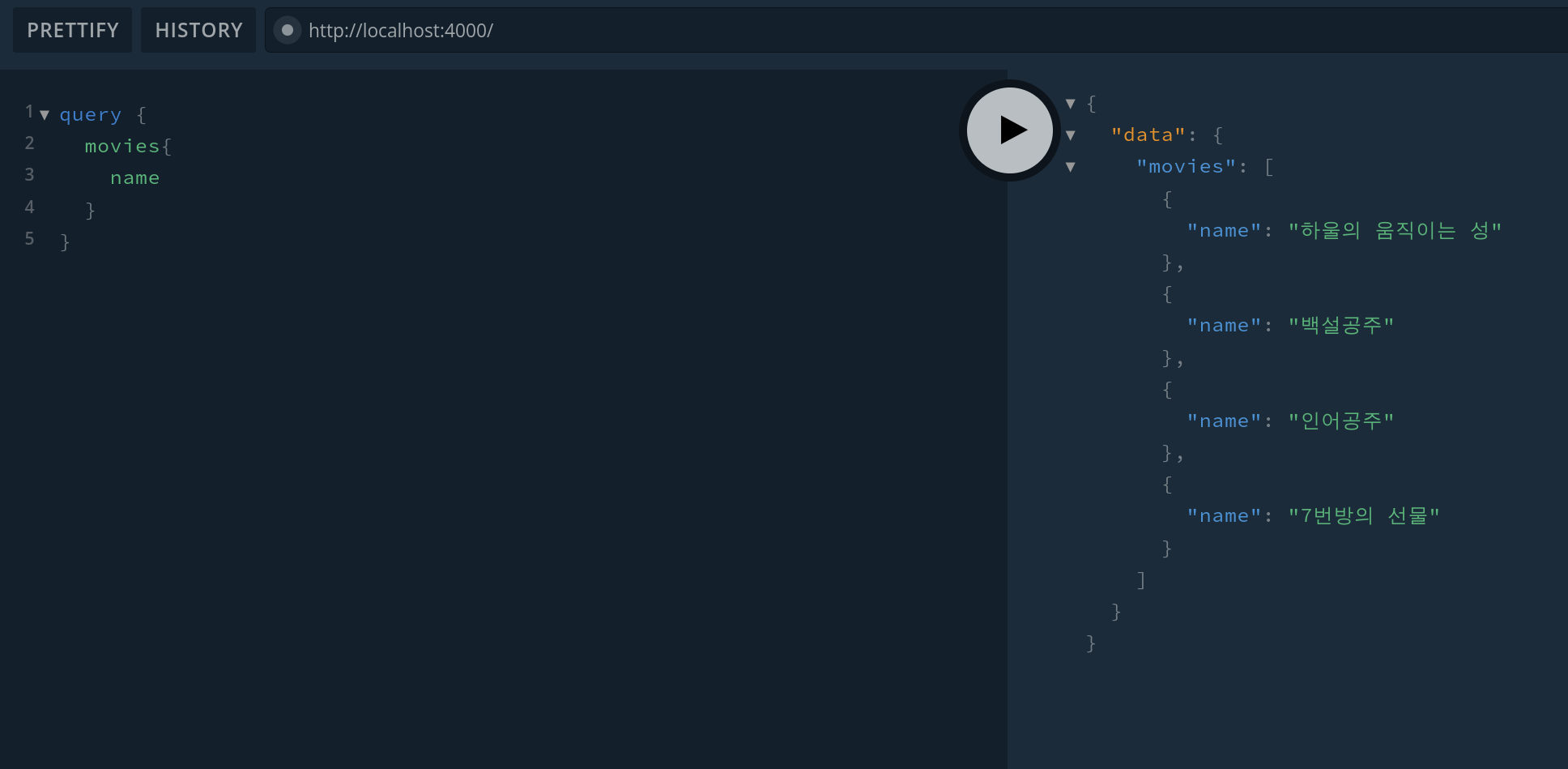
mutation은 CRUD에서 CUD기능을 담당한다.