Linked List
Linked List๐คฎ
์ฌ์ ์ง์
-
Storage โก๏ธ (HDD/SSD) ๊ฐ๊ฒฉ์ด ์ ๋ ดํ๋ฉฐ ์ฉ๋์ด ํฌ๊ณ ์ ์์ด ๊บผ์ ธ๋ ๋ฐ์ดํฐ๊ฐ ์ ์ฅ๋จ
-
Memory โก๏ธ (DRAM) ๊ฐ๊ฒฉ์ด ๋น์ธ๊ณ ์ฉ๋์ด ์ ๊ณ ์ ์์ด ๊บผ์ง๋ฉด ๋ฐ์ดํฐ๊ฐ ์ฌ๋ผ์ง But ์๋๊ฐ ๋น ๋ฅด๋ค
-
CPU โก๏ธ ๋ฐ์ดํฐ๋ฅผ ๋์ด๋ค ์ธ๋ Storage๊ฐ ์๋ Memory์์ ๋ฐ์ดํฐ๋ฅผ ๋์ด๋ค ์ ( Memory๊ฐ ์๋๊ฐ ๋น ๋ฅด๊ธฐ ๋๋ฌธ )
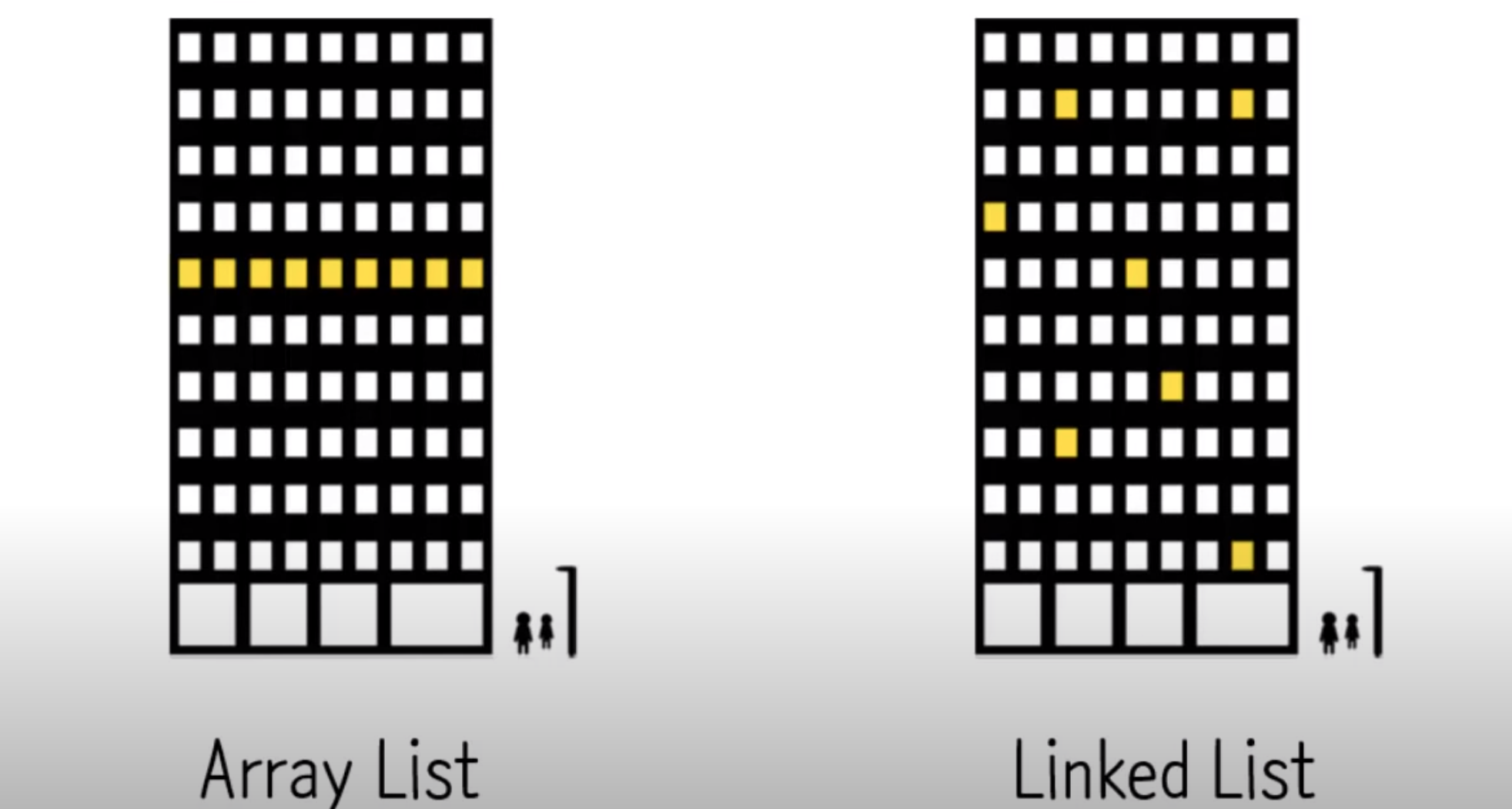
Array List โก๏ธ [์ถ๊ฐ/์ญ์ : ๋๋ฆผ ( ๋ฉ๋ชจ๋ฆฌ์ฃผ์๋ฅผ ํ์นธํ์นธ์ฉ ๋ก๊ฒจ์ผํ๊ธฐ๋๋ฌธ )] [์ธ๋ฑ์ค ์กฐํ: ๋น ๋ฆ ( ์ฃผ์๋ฅผ ์๊ณ ์์ )]
Linked List โก๏ธ [์ถ๊ฐ/์ญ์ : ๋น ๋ฆ ( ์ฐ๊ฒฐ๋ง ์ฌ์ค์ ํด์ฃผ๋ฉด๋๊ธฐ๋๋ฌธ )] [์ธ๋ฑ์ค ์กฐํ: ๋๋ฆผ ( ํ๋ํ๋ ํ๊ณ ์กฐํํด์ผํจ )]
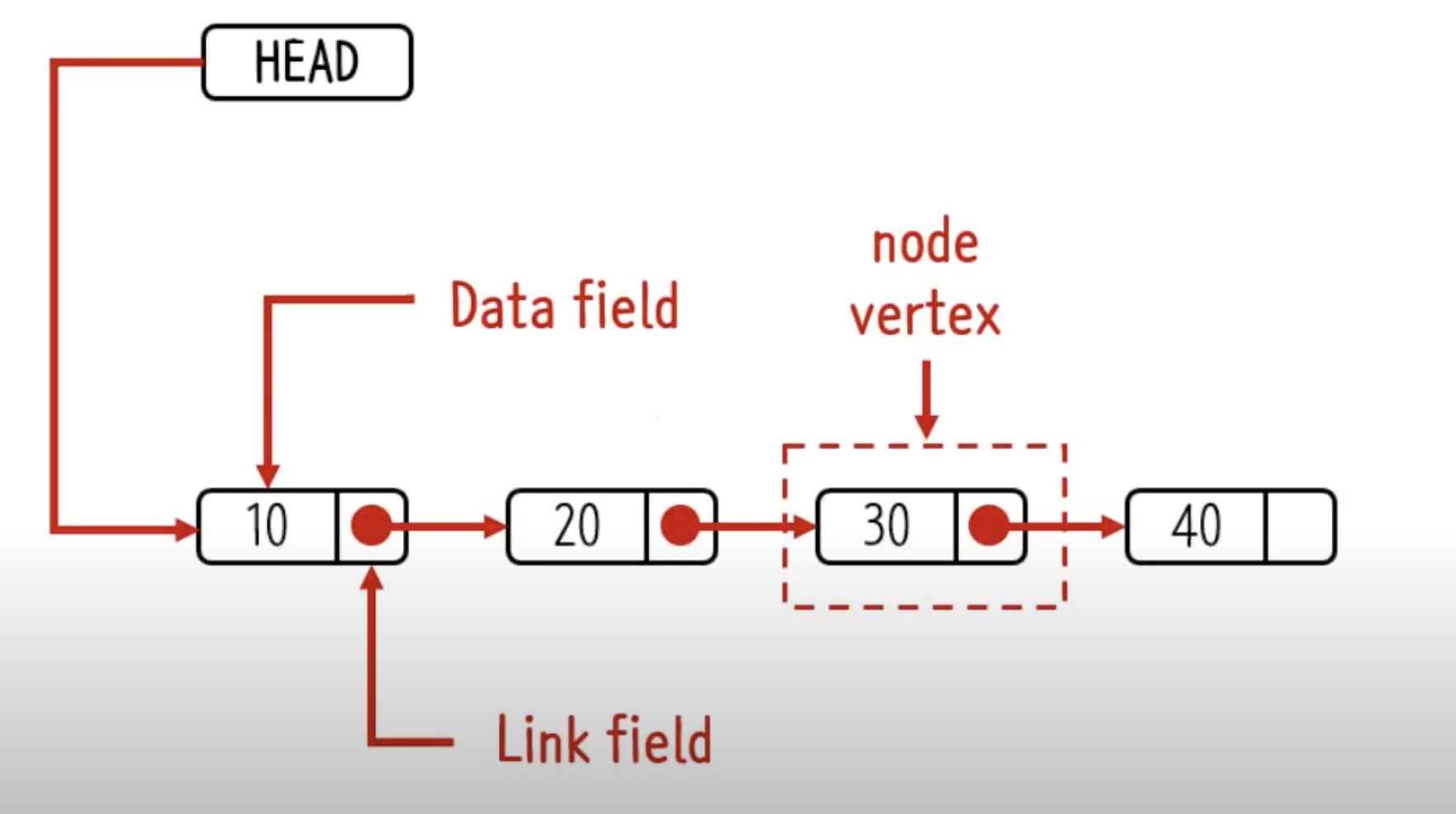
-
node โก๏ธ dataField + linkField ๊ทธ๋ฆผ์์๋ ์ด 4๊ฐ์ node๊ฐ ์กด์ฌํจ (JS๋ ๊ฐ์ฒด์งํฅ์ด๋ฏ๋ก ์ด๊ฒ์ ๊ฐ์ฒด๋ก ํํ)
-
data field โก๏ธ ์ค์ ๊ฐ
-
link field โก๏ธ ๋ค์ node๊ฐ ๋ฌด์์ธ๊ฐ์ ๋ํด ์ ์ฅ๋์ด์์
-
Head โก๏ธ ์ฒซ๋ฒ์ฉจ node๊ฐ ๋ฌด์์ธ๊ฐ์ ๋ํด ์ ์ฅ๋์ด์์
์ค์ ๋ก ๋ง๋ค์ด๋ณด๊ธฐ
test.js
const LinkedList = function () {
this.head = null;
this.size = 0;
};
const Node = function (data) {
this.data = data;
this.link = null;
};
์์ผ๋ก ์ฐ์ด๋ผ ๊ฐ์ฒด๋ฅผ ๋ง๋ค ํจ์๋ค
LinkedList.prototype.add = function (data) {
const newNode = new Node(data);
if (this.head === null) {
this.head = newNode; // Node { data: 5, link: null } // ํค๋์ ์ฃผ์๋ฃ์ด์ฃผ๊ธฐ
this.size++;
} else {
let currentNode = this.head;
while (currentNode.link !== null) {
currentNode = currentNode.link;
}
currentNode.link = newNode;
this.size++;
}
};
์์ฑ์์ ์ํด ์์ฑ๋ ๊ฐ์ฒด๊ฐ ์ฌ์ฉํ ์ ์๊ฒ ํ๋กํ ํ์ ์ผ๋ก add ํจ์ ๊ตฌํ
add() โก๏ธ ๋งํฌ๋ฆฌ์คํธ์ ๊ฐ์ ๋ฃ์ด์ฃผ๋ ํจ์๋ก ๋์์ธํจ
-
๋ ธ๋๊ฐ์ฒด๋ฅผ ์์ฑ ํ๋ค.
-
head๊ฐ์ด null์ด๋ผ๋ฉด head์ ์๋ก ์์ฑํ ๋ ธ๋๋ฅผ ๋ฃ๋๋ค.
-
head๊ฐ์ด ์๋ค๋ฉด ๋งํฌ๋ฅผ ํ๊ณ ํ๊ณ ๋ค์ด๊ฐ ๋งํฌ๊ฐ์ด null์ผ๋, ์ฆ ๋ง์ง๋ง ๋ ธ๋์ ๊ฐ์ ์๋ก ์์ฑ๋ ๋ ธ๋๋ฅผ ๋ฃ๋๋ค.
LinkedList.prototype.insertFirstNode = function (data) {
const newNode = new Node(data);
newNode.link = this.head;
this.head = newNode;
this.size++;
};
insertFirstNode() โก๏ธ ์ฒซ๋ฒ์งธ๋ ธ๋์ โ๊ฐ์ด ์ถ๊ฐ๋๋๋กโ ๋์์ธํจ
-
๋ ธ๋๊ฐ์ฒด๋ฅผ ์์ฑ ํ๋ค.
-
์์ฑ๋ ๋ ธ๋๊ฐ์ฒด์ ๋งํฌ๊ฐ์ผ๋ก ํ์ฌ์ head๊ฐ์ ๋ฃ์ด์ค๋ค.
-
ํ์ฌ์ head๊ฐ์ ์๋ก์ด ๋ ธ๋๊ฐ์ฒด์ ๊ฐ์ผ๋ก ๋์ฒด๋์ด์ง๋ค.
LinkedList.prototype.insertMiddleNode = function (data, index) {
const newNode = new Node(data);
let beforeNode = this.head;
while (--index !== 0) {
beforeNode = beforeNode.link;
}
let afterNode = beforeNode.link;
beforeNode.link = newNode;
newNode.link = afterNode;
this.size++;
};
insertMiddleNode() โก๏ธ ์ฒซ๋ฒ์งธ ๋ ธ๋๋ฅผ ์ ์ธํ ์ด๋๊ณณ์์๋ โ๊ฐ์ด ์ถ๊ฐ๋๋๋กโ ๋์์ธํจ
-
๋ ธ๋๊ฐ์ฒด๋ฅผ ์์ฑ ํ๋ค.
-
beforeNode๋ฅผ ์์ฑํ์ฌ ํ์ฌ head์ ๊ฐ์ ๋ฃ์ด์ค๋ค.
-
์ ๋ฌ๋ฐ์ index๊ฐ์ ํตํด ๊ฐ์ด ์ถ๊ฐ๋์ด์ผํ ์๋ฆฌ์ ๋ฐ๋ก ์ ๋ ธ๋๊ฐ์ฒด๋ฅผ beforeNode์ ๋ฃ์ด์ค๋ค.
-
beforeNode์๋ฅผ ํตํด ์์๋ธ ๊ทธ ๋ค์ ๋ ธ๋๊ฐ์ฒด๋ฅผ ์๋ก์์ฑํ afterNode์ ์ ์ฅํ๋ค.
-
๊ฐ ๋งํฌ๋ค์ ์๋ง๊ฒ ์ฌ์ ์ํด์ค๋ค.
LinkedList.prototype.delete = function (index) {
let beforeNode = this.head;
if (index >= 1) {
while (--index !== 0) {
beforeNode = beforeNode.link;
}
let tobeDelete = beforeNode.link;
let afterNode = beforeNode.link.link;
beforeNode.link = afterNode;
delete tobeDelete;
} else {
let tobeDelete = beforeNode;
let afterNode = beforeNode.link;
this.head = afterNode;
delete tobeDelete;
}
this.size--;
};
delete() โก๏ธ ์ด๋๊ณณ์ด๋ ์๊ด์์ด ๋ ธ๋๋ฅผ ์ญ์ ํ๊ณ ์ฌ์ ์ํ๊ฒ ๋์์ธํจ.
-
index๊ฐ์ด 1 ์ด์์ด๋ผ๋ฉด ๋ ธ๋๋ฅผ ์ฌ์ ์ํด์ค๋ค.
-
index๊ฐ์ด ๊ทธ ์ธ ๋ผ๋ฉด head๋ฅผ ์ฌ์ ์ํด์ค๋ค.
LinkedList.prototype.showData = function () {
let currentNode = this.head;
const result = Array(this.size).fill(0);
for (let i = 0; i < this.size; i++) {
result[i] = currentNode.data;
currentNode = currentNode.link;
}
return result;
};
showData() โก๏ธ ๋งํฌ๋ฆฌ์คํธ๋ฅผ ๋ฐฐ์ด๋ก ์ฌ์ ์ํ์ฌ ๋ณผ ์ ์๊ฒ ๋์์ธํจ.
-
fill(value, start, end) ex) fill(5,0,3) 5๋ผ๋ ์ซ์๋ก 0~3์ ์ธ๋ฑ์ค๊น์ง ์ฑ์์ฃผ์ .
-
์ฆ fill(0) 0์ด๋ผ๋ ์ซ์๋ก ์ ๋ถ๋ค ์ฑ์์ฃผ์ .
const a = new LinkedList();
a.add(1); // [1]
a.add(5); // [1,5]
a.add(4); // [1,5,4]
a.insertMiddleNode(3, 2); // [1,5,3,4]
a.delete(0); // [5,3,4]
console.log(a.showData());
๊ฒฐ๊ณผ๋ ์ฑ๊ณต์ .
class ๋ฌธ๋ฒ์ผ๋ก ์ฝ๋์นดํ ํ์ด๋ณด๊ธฐ
class Node {
constructor(val) {
this.val = val;
this.next = null;
}
}
class MyLinkedList {
constructor() {
this.head = null;
this.tail = null;
this.size = 0;
}
addAtHead(val) {
const newNode = new Node(val);
if (this.head === null) {
this.head = newNode;
} else {
newNode.next = this.head;
this.head = newNode;
}
this.size++;
}
addAtTail(val) {
const newNode = new Node(val);
if (this.tail === null) {
this.tail = newNode;
let currentNode = this.head;
while (currentNode.next !== null) {
currentNode = currentNode.next;
}
currentNode.next = this.tail;
} else {
let last = this.tail;
last.next = newNode;
this.tail = newNode;
}
this.size++;
}
addAtIndex(index, val) {
if (index <= this.size - 1) {
const newNode = new Node(val);
let beforeNode = this.head;
while (--index !== 0) {
beforeNode = beforeNode.next;
}
let afterNode = beforeNode.next;
beforeNode.next = newNode;
newNode.next = afterNode;
this.size++;
} else {
return;
}
}
deleteAtIndex(index) {
let beforeNode = this.head;
if (index >= 1) {
while (--index !== 0) {
beforeNode = beforeNode.next;
}
let afterNode = beforeNode.next.next;
delete beforeNode.next;
beforeNode.next = afterNode;
} else {
let afterNode = beforeNode.next;
delete beforeNode.next;
this.head = afterNode;
}
this.size--;
}
get(index) {
let currentNode = this.head;
while (index !== 0) {
if (currentNode.next) {
currentNode = currentNode.next;
} else {
return -1;
}
index--;
}
return currentNode.val;
}
}
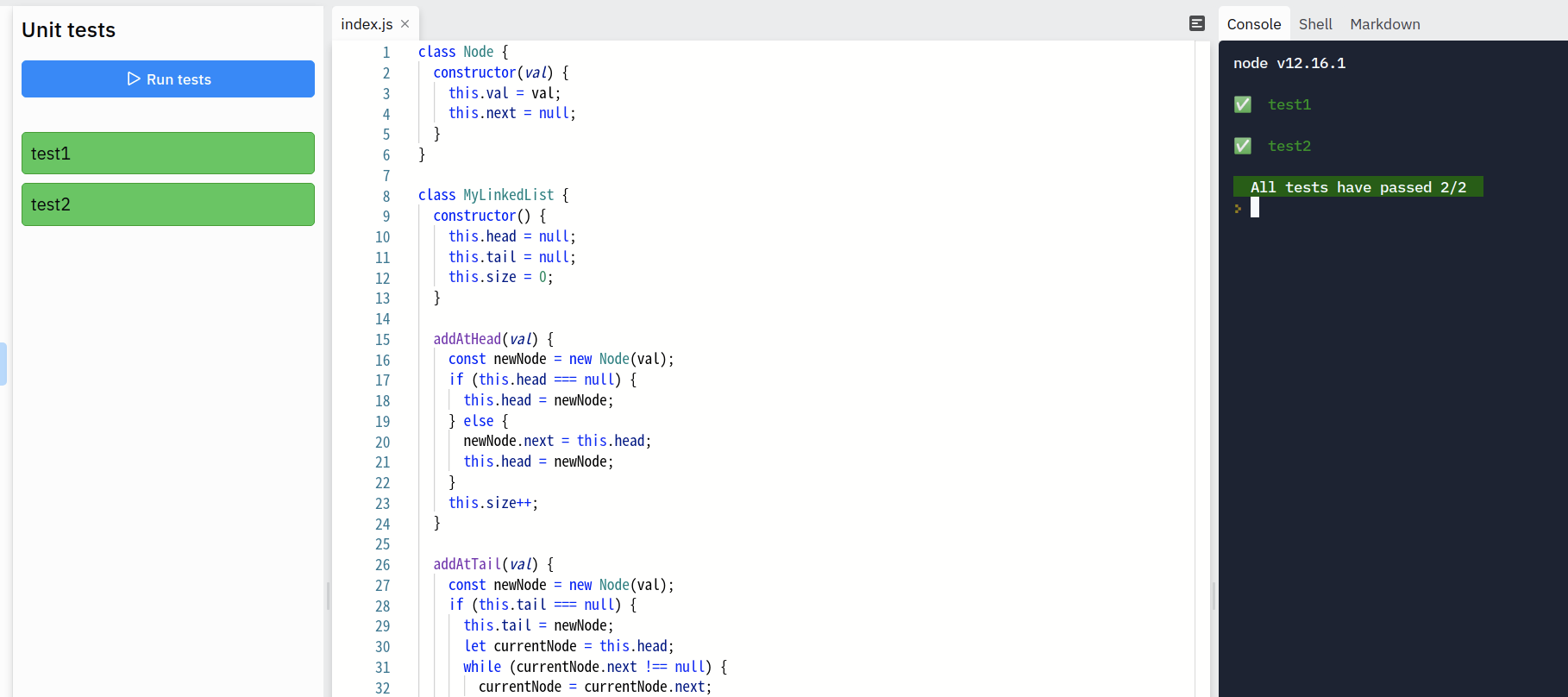
์ฑ๊ณต!!