프로그래머스 키패드 누르기
Algorithm🤮
이문제는 접근을 어떻게할지 몰라 접근하는데만 30분을 소비했다… 하지만 이문제를 풀면서 JS의 기초를 좀 더 쌓을 수 있었기에 리뷰한다.
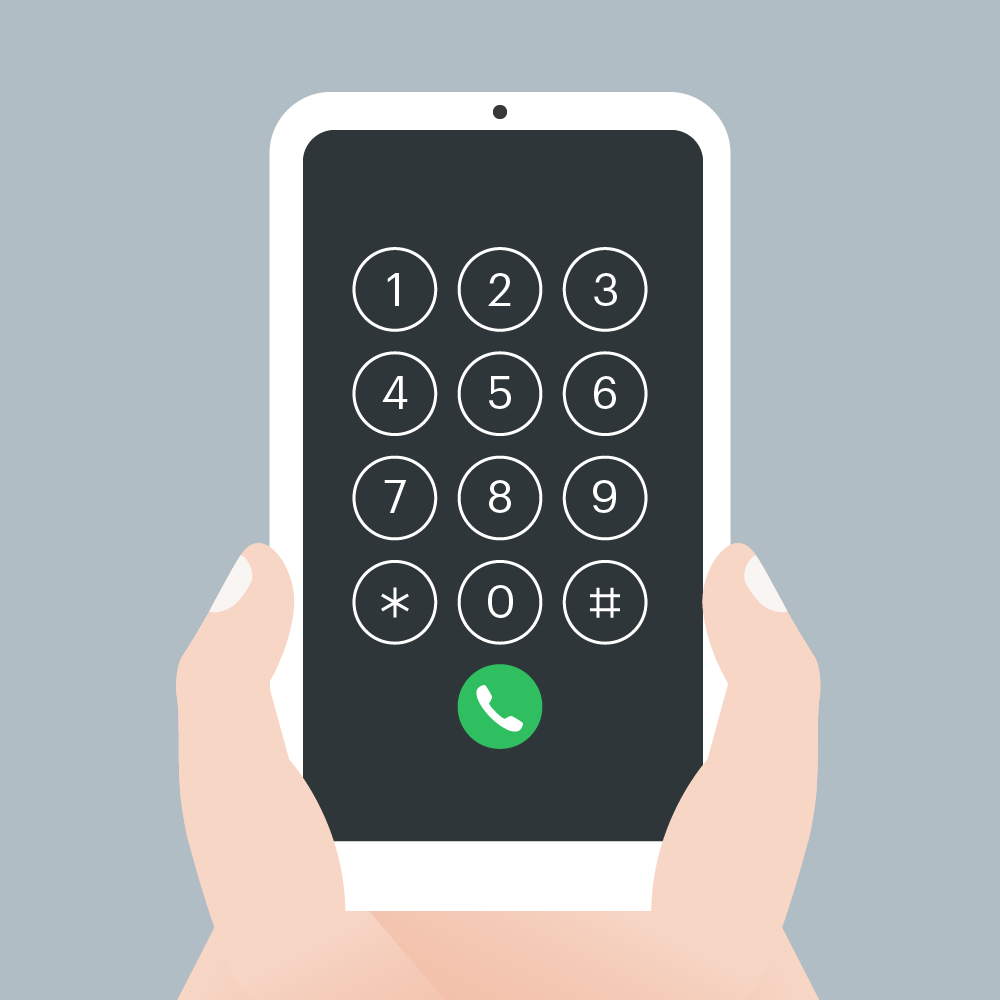
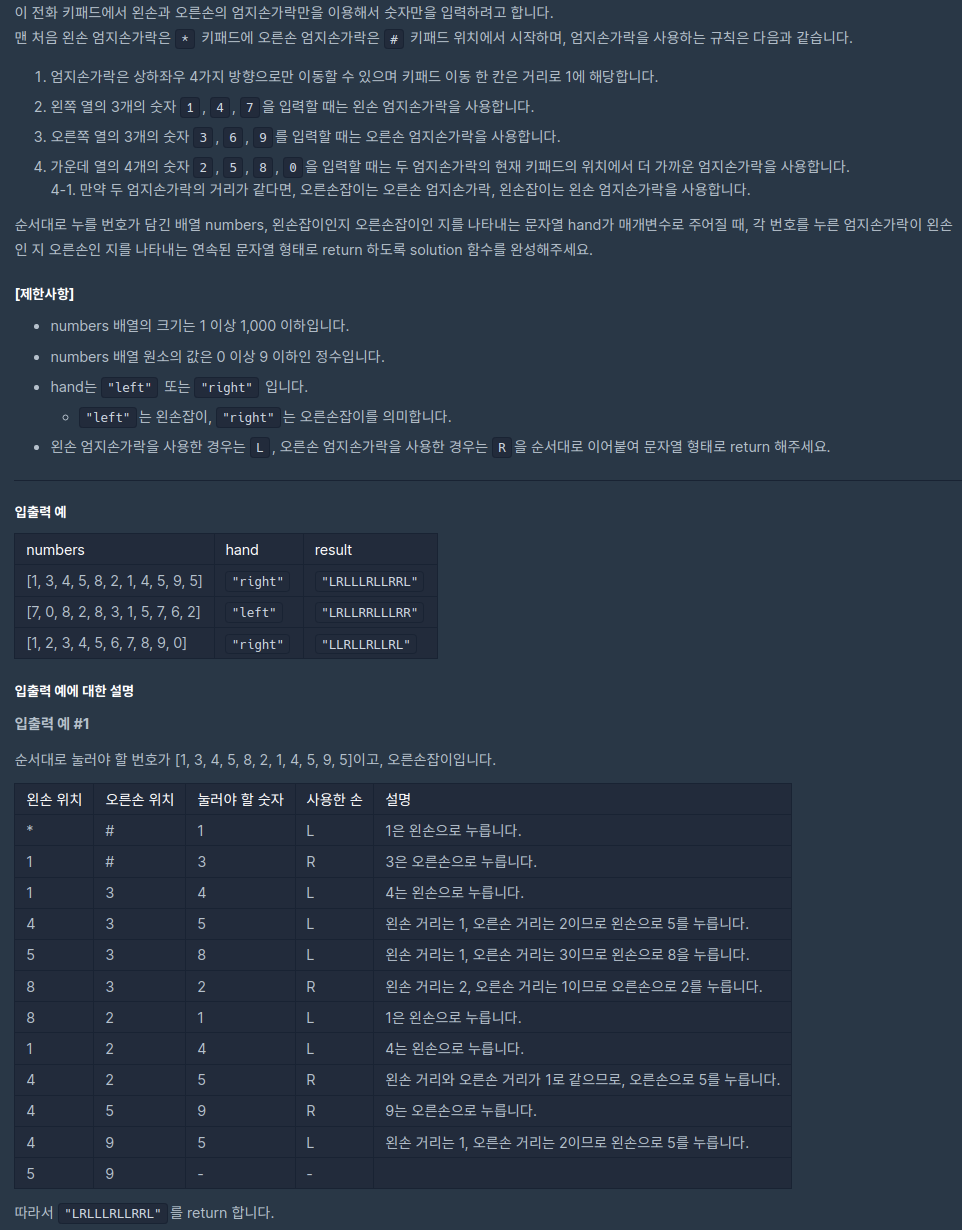
function solution(numbers, hand) {
let result = [];
let left = [];
let right = [];
let distance = [
{ 0: 0, 8: 1, 579: 2, 246: 3, 13: 4 },
1,
{ 2: 0, 135: 1, 486: 2, 790: 3 },
3,
4,
{ 5: 0, 2468: 1, 13790: 2 },
6,
7,
{ 8: 0, 5790: 1, 246: 2, 13: 3 },
{ 0: 1, 8: 2, 5: 3, 2: 4 },
];
numbers.forEach((e, i) => {
if (e === 7 || e === 4 || e === 1) {
result[i] = "L";
left[i] = [e];
} else if (e === 9 || e === 6 || e === 3) {
result[i] = "R";
right[i] = [e];
} else {
let leftDistance;
let rightDistance;
if (left.length === 0 || right.length === 0) {
if (left.length === 0) {
for (let val in distance[e]) {
if (val.includes(right[right.length - 1])) {
rightDistance = distance[e][val];
}
}
if (distance[9][e] < rightDistance) {
result[i] = "L";
left[i] = [e];
} else if (distance[9][e] > rightDistance) {
result[i] = "R";
right[i] = [e];
} else {
if (hand === "left") {
result[i] = "L";
left[i] = [e];
} else {
result[i] = "R";
right[i] = [e];
}
}
}
if (right.length === 0) {
for (let val in distance[e]) {
if (val.includes(left[left.length - 1])) {
leftDistance = distance[e][val];
}
}
if (distance[9][e] < leftDistance) {
result[i] = "R";
right[i] = [e];
} else if (distance[9][e] > leftDistance) {
result[i] = "L";
right[i] = [e];
} else {
if (hand === "left") {
result[i] = "L";
left[i] = [e];
} else {
result[i] = "R";
right[i] = [e];
}
}
}
} else {
for (let val in distance[e]) {
if (val.includes(left[left.length - 1])) {
leftDistance = distance[e][val];
}
}
for (let val in distance[e]) {
if (val.includes(right[right.length - 1])) {
rightDistance = distance[e][val];
}
}
if (leftDistance < rightDistance) {
result[i] = "L";
left[i] = [e];
} else if (leftDistance > rightDistance) {
result[i] = "R";
right[i] = [e];
} else {
if (hand === "left") {
result[i] = "L";
left[i] = [e];
} else {
result[i] = "R";
right[i] = [e];
}
}
}
}
});
return result.join("");
}
코드가 상당히 더럽지만 요약하자면
우선 0,2,5,8 키에서의 다른번호의 키로 가는 거리값을 배열에 저장시켰다.
그리고 1,4,7은 왼손으로 누르고 그값을 left변수에 저장, 마찬가지로 3,6,9는 오른손으로 누르고 그값을 right변수에 저장
만약 left or right에 값이 들어가있지않다면 (=> 처음부터 0,2,5,8 중하나를 누름)
값이 없는 변수와 값이 들어가있는 left of right distance를 비교한다.
또한 왼손인지 오른손인지 또한 비교한다.
끝.